Render 3D objects in OpenGL ES
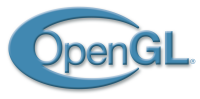
So you created a gorgeous 3D model in Cinema4D and want to have it rendered in an iPhone app? And it should be able to rotate and zoom the object by handling touches? Look no further and enjoy.
I was looking for such a solution and stumbled upon mtl2opengl, a Perl script to convert a 3D model into a header file which can easily be used in a OpenGL/ES app. Ricardo even provided an Xcode boilerplate app to demonstrate this.
I then enhanced the code to handle touches and pinches in order to allow for rotation and zooming and the result is fabulous.
Now, for the technical part, this is the code which I added in order to support rotation and zooming. Not that hard, huh?
[objc] - (void)viewDidLoad { //.. added a pinch gesture recognizer UIPinchGestureRecognizer* pinch = [[UIPinchGestureRecognizer alloc] initWithTarget:self action:@selector(pinched:)]; [self.view addGestureRecognizer:pinch]; } -(void)pinched:(UIPinchGestureRecognizer*)sender { if([(UIPinchGestureRecognizer*)sender state] == UIGestureRecognizerStateEnded) { scale = 1.0; lastScale = 1.0; } else { scale = scale - (lastScale - sender.scale); lastScale = sender.scale; } } -(void)touchesMoved:(NSSet *)touches withEvent:(UIEvent *)event { UITouch* t = [touches anyObject]; rotation.x += ([t locationInView:self.view].x - [t previousLocationInView:self.view].x); rotation.y += ([t locationInView:self.view].y - [t previousLocationInView:self.view].y); } - (void)updateViewMatrices { //.. adapt the model view matrix with rotation and zoomscale _modelViewMatrix = GLKMatrix4MakeTranslation(0.0, 0.0, -0.6); _modelViewMatrix = GLKMatrix4Rotate(_modelViewMatrix, GLKMathDegreesToRadians(rotation.y), 1.0, 0.0, 0.0); _modelViewMatrix = GLKMatrix4Rotate(_modelViewMatrix, GLKMathDegreesToRadians(rotation.x), 0.0, 1.0, 0.0); _modelViewMatrix = GLKMatrix4Scale(_modelViewMatrix, 0.4 * scale, 0.4 * scale, 0.4 * scale); } [/objc]
You can download a copy of the adapted code on Github
- Log in to post comments